Connect to Redis™ from Your Application
Once your ScaleGrid for Redis™ deployment is set up, you can easily connect to your new deployment via your application. Follow these instructions to connect to Redis™ from your application
Firewall rules
In order for the source machine to connect to the cluster, you need to create a firewall rule to allow it to connect. Please refer to this help doc.
Collect the information from GUI
You need the following info at hand when connecting, this can be found under the Overview tab on the cluster details page in the ScaleGrid console:
- Hostname: The Connection String is in the format [hostname:port], and the hostname looks something like this: SG-redms-17-redis-dev.testserver.scalegrid.io
- Port: Default port is 6379 for Redis™
- Password: You can get and reset your password from the GUI
- SSL Certificates: For SSL enabled clusters, you will also need the SSL CA certificate file. Download and save the file to a location on the server on which the code will be deployed
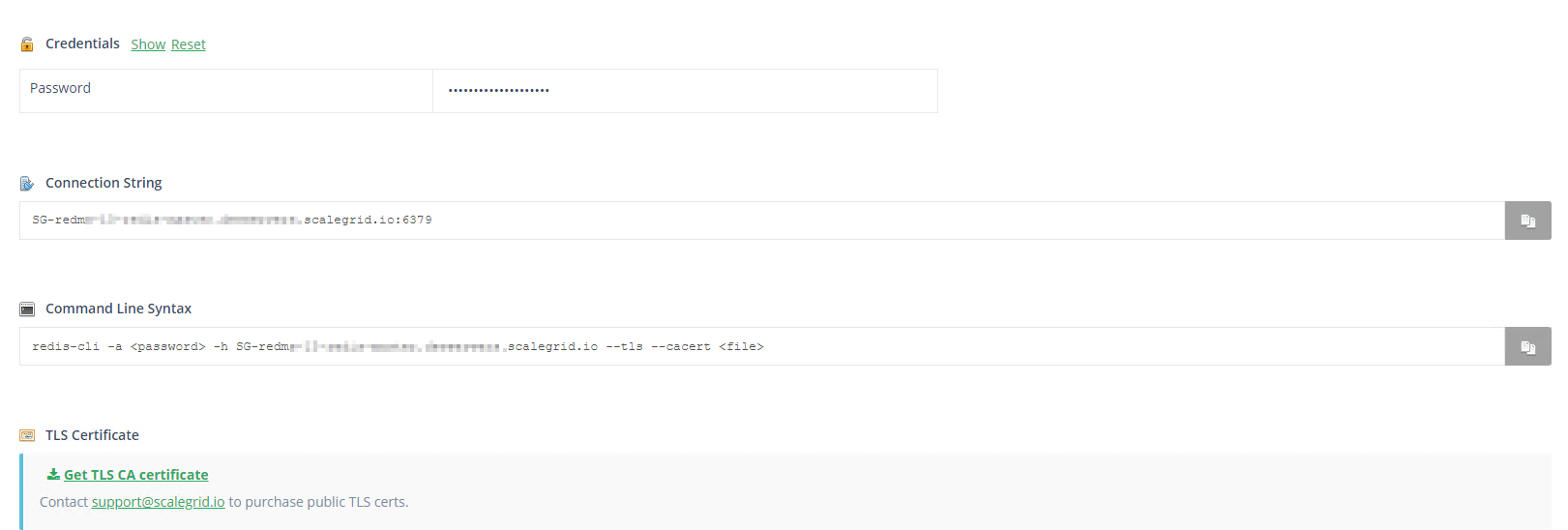
Platform-Specific Code Snippets to connect to your Redis™ cluster
Here are a few sample code snippets for you to use when connecting to your cluster.
Remember to replace <YOUR_HOSTNAME>, <YOUR_PASSWORD>, and (if you are connecting with SSL) 'LOCAL/PATH/TO/SSLcert.pem' in each sample snippet to match your specific info.
Python
If you need to install redis-py, simply run the following command:
$ pip install redis
Here are the code samples for connecting with Python:
import redis
client=redis.Redis(host='<YOUR_HOSTNAME>', port=6379, password='<YOUR_PASSWORD>', ssl=True, ssl_ca_certs= 'LOCAL/PATH/TO/SSLcert.pem')
#some testing commands
client.set('foo','bar')
print(client.get('foo'))
print(client.dbsize())
import redis
client=redis.Redis(host='<YOUR_HOSTNAME>', port=6379, password='<YOUR_PASSWORD>')
#some testing commands
client.set('foo','bar')
print(client.get('foo'))
print(client.dbsize())
You can refer to this documentation for more information: Redis-py
Ruby
If you need to install redis-rb, simply run the following command:
$ gem install redis
Here are the code samples for connecting with Ruby:
require 'redis'
begin
redis = Redis.new(
:host => '<YOUR_HOSTNAME>',
:port => 6379,
:password => '<YOUR_PASSWORD>',
:ssl => :true,
:ssl_params => {
:ca_file => 'LOCAL/PATH/TO/SSLcert.pem'
}
)
puts redis.info
client_ping = redis.ping
if client_ping
puts 'Connected!'
else
raise 'Ping failed'
end
rescue => e
puts "Error: #{e}"
end
require 'redis'
begin
redis = Redis.new(
:host => '<YOUR_HOSTNAME>',
:port => 6379,
:password => '<YOUR_PASSWORD>'
)
puts redis.info
client_ping = redis.ping
if client_ping
puts 'Connected!'
else
raise 'Ping failed'
end
rescue => e
puts "Error: #{e}"
end
Node.js (node_redis)
If you need to install node_redis, all you need to do is to run the following command:
$ npm install redis
Here are the code samples for connecting with node_redis:
const redis = require('redis');
const fs = require('fs');
const client = redis.createClient({
host: '<YOUR_HOSTNAME>',
port: 6379,
password: '<YOUR_PASSWORD>',
tls: {
ca: [ fs.readFileSync('LOCAL/PATH/TO/SSLcert.pem', encoding='ascii') ]
}
});
client.on('error', err => {
console.log('Error ' + err);
});
client.set('foo', 'bar', (err, reply) => {
if (err) throw err;
console.log(reply);
client.get('foo', (err, reply) => {
if (err) throw err;
console.log(reply);
});
});
const redis = require('redis');
const fs = require('fs');
const client = redis.createClient({
host: '<YOUR_HOSTNAME>',
port: 6379,
password: '<YOUR_PASSWORD>'
});
client.on('error', err => {
console.log('Error ' + err);
});
client.set('foo', 'bar', (err, reply) => {
if (err) throw err;
console.log(reply);
client.get('foo', (err, reply) => {
if (err) throw err;
console.log(reply);
});
});
Node.js (ioredis)
If you need to install node_redis, all you need to do is to run the following command:
$ npm install ioredis
Here are the code samples for connecting with ioredis:
const Redis = require('ioredis');
const fs = require('fs');
const redis = new Redis({
host: '<YOUR_HOSTNAME>',
port: 6379,
password: '<YOUR_PASSWORD>',
tls: {
ca: [ fs.readFileSync('LOCAL/PATH/TO/SSLcert.pem', 'ascii') ]
}
});
redis.info().then(function (result) {
console.log(result);
});
const Redis = require('ioredis');
const fs = require('fs');
const redis = new Redis({
host: '<YOUR_HOSTNAME>',
port: 6379,
password: '<YOUR_PASSWORD>'
});
redis.info().then(function (result) {
console.log(result);
});
JAVA (jedis)
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
import redis.clients.jedis.exceptions.JedisException;
import redis.clients.jedis.Protocol;
import java.io.InputStream;
import java.io.FileInputStream;
import java.net.Socket;
import java.security.KeyStore;
import java.security.cert.CertificateFactory;
import java.security.cert.X509Certificate;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSocketFactory;
import javax.net.ssl.TrustManager;
import javax.net.ssl.TrustManagerFactory;
public class TestConnectionWithSSL {
private static final Integer redisPort = 6379;
private static final String redisHost = "<hostname>";
private static final String password = "<YOUR_PASSWORD>";
private static final String certFile = "LOCAL/PATH/TO/SSLcert.pem";
private static final String certName = "ScaleGrid-db-cert";
private static JedisPool pool = null;
public TestConnectionWithSSL() throws Exception {
JedisPoolConfig config = new JedisPoolConfig();
System.out.println("JedisPool timeout value " + Protocol.DEFAULT_TIMEOUT + " ms");
pool = new JedisPool(config, redisHost, redisPort, Protocol.DEFAULT_TIMEOUT, password, true, getTlsSocketFactory(), null, null);
}
public void set(String key, String value) throws Exception {
Jedis jedis = null;
jedis = pool.getResource();
Socket socket = jedis.getClient().getSocket();
System.out.println("Connected to " + socket.getRemoteSocketAddress());
jedis.set(key, value);
}
public String get(String key) throws Exception {
Jedis jedis = null;
jedis = pool.getResource();
return jedis.get(key);
}
private SSLSocketFactory getTlsSocketFactory() throws Exception {
SSLContext sslContext = SSLContext.getInstance("TLSv1.2");
KeyStore trustStore = KeyStore.getInstance(KeyStore.getDefaultType());
trustStore.load(null, null);
// Get the cert
X509Certificate result;
InputStream input = new FileInputStream(certFile);
result = (X509Certificate) CertificateFactory.getInstance("X509").generateCertificate(input);
// Add cert to the trust store
trustStore.setCertificateEntry(certName, result);
TrustManagerFactory tmf = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
tmf.init(trustStore);
TrustManager[] trustManagers = tmf.getTrustManagers();
sslContext.init(null, trustManagers, null);
return sslContext.getSocketFactory();
}
public static void main(String[] args) throws Exception {
TestConnectionWithSSL main = new TestConnectionWithSSL();
main.set("test","test");
System.out.println("test: " + main.get("test"));
}
import redis.clients.jedis.Jedis;
import redis.clients.jedis.JedisPool;
import redis.clients.jedis.JedisPoolConfig;
import redis.clients.jedis.exceptions.JedisException;
import redis.clients.jedis.Protocol;
import java.net.Socket;
public class TestConnection {
//address of your redis server
private static final Integer redisPort = 6379;
private static final String redisHost = "<YOUR_HOSTNAME>";
private static final String password = "<YOUR_PASSWORD>";
//the jedis connection pool..
private static JedisPool pool = null;
public TestConnection() {
//configure our pool connection
JedisPoolConfig config = new JedisPoolConfig();
System.out.println("JedisPool timeout value " + Protocol.DEFAULT_TIMEOUT + " ms");
pool = new JedisPool(config, redisHost, redisPort, Protocol.DEFAULT_TIMEOUT, password);
}
public void set(String key, String value) throws Exception {
Jedis jedis = null;
jedis = pool.getResource();
Socket socket = jedis.getClient().getSocket();
System.out.println("Connected to " + socket.getRemoteSocketAddress());
jedis.set(key, value);
}
public String get(String key) throws Exception {
Jedis jedis = null;
jedis = pool.getResource();
return jedis.get(key);
}
public static void main(String[] args) throws Exception {
TestConnection main = new TestConnection();
main.set("test","test");
System.out.println("test: " + main.get("test"));
}
}
Go
If you need to install Go, visit their website and follow the instructions.
Create a main.go using the connection snippets below, then simply run the following commands:
$ /usr/local/go/bin/go mod init main.go
$ /usr/local/go/bin/go get github.com/gomodule/redigo/redis
$ /usr/local/go/bin/go run main.go
package main
import (
"fmt"
"log"
"github.com/gomodule/redigo/redis"
)
func main() {
// Connect to Redis with SSL Enabled
conn, err := redis.Dial("tcp", "<YOUR_HOSTNAME>:6379", redis.DialPassword("<YOUR_PASSWORD>"), redis.DialUseTLS(true), redis.DialTLSSkipVerify(true)
)
if err != nil {
log.Fatal(err)
}
// Importantly, use defer to close the connection before exiting the main() function.
defer conn.Close()
_, err = conn.Do("SET", "testgo1", "1")
if err != nil {
log.Fatal(err)
}
value, err := redis.String(conn.Do("GET", "testgo1"))
if err != nil {
log.Fatal(err)
}
fmt.Println("GET testgo1 " + value)
}
package main
import (
"fmt"
"log"
"github.com/gomodule/redigo/redis"
)
func main() {
// Connect to Redis with no SSL
conn, err := redis.Dial("tcp", "<YOUR_HOSTNAME>:6379", redis.DialPassword("<YOUR_PASSWORD>"))
if err != nil {
log.Fatal(err)
}
// Importantly, use defer to close the connection before exiting the main() function.
defer conn.Close()
_, err = conn.Do("SET", "testgo1", "1")
if err != nil {
log.Fatal(err)
}
value, err := redis.String(conn.Do("GET", "testgo1"))
if err != nil {
log.Fatal(err)
}
fmt.Println("GET testgo1 " + value)
}
Django
If you need to install Django, you will need to use django-redis. Install it by using the following command:
$ pip install django-redis
In your settings.py, configure your CACHES like this:
CACHES = {
"default": {
"BACKEND": "django_redis.cache.RedisCache",
"LOCATION": "rediss://<YOUR_HOSTNAME>:6379",
"OPTIONS": {
"CLIENT_CLASS": "django_redis.client.DefaultClient",
"PASSWORD": "<YOUR_PASSWORD>",
'REDIS_CLIENT_KWARGS': {
'ssl': True,
'ssl_cert_reqs': None
}
},
}
}
CACHES = {
"default": {
"BACKEND": "django_redis.cache.RedisCache",
"LOCATION": "redis://<YOUR_HOSTNAME>:6379",
"OPTIONS": {
"CLIENT_CLASS": "django_redis.client.DefaultClient",
"PASSWORD": "<YOUR_PASSWORD>"
}
}
PHP
To use Redis™ in your PHP application, you will need to use Predis. This library can be found on Packagist for easier management of project dependencies using Composer. Compressed archives of each release are available on GitHub.
To load the library from the compressed release files, use the following commands:
$ wget https://github.com/predis/predis/archive/refs/tags/v1.1.10.zip
$ mv predis-1.1.10 predis
<?php
require "predis/autoload.php";
Predis\Autoloader::register();
$redis = new Predis\Client(array(
"scheme" => "tcp",
"host" => "<YOUR_HOSTNAME>",
"port" => 6379,
'scheme' => 'tls',
'ssl' => ['cafile' => 'LOCAL/PATH/TO/SSLcert.pem', 'verify_peer' => true],
"password" => "<YOUR_PASSWORD>"));
echo "Connected to Redis";
$redis->set("foo", "bar");
$value = $redis->get("foo");
var_dump($value);
?>
<?php
require "predis/autoload.php";
Predis\Autoloader::register();
$redis = new Predis\Client(array(
"scheme" => "tcp",
"host" => "<YOUR_HOSTNAME>",
"port" => 6379,
"password" => "<YOUR_PASSWORD>"));
echo "Connected to Redis";
$redis->set("foo", "bar");
$value = $redis->get("foo");
var_dump($value);
?>
.NET
To use Redis™ in your .NET application, you will need to use StackExchange.Redis, a general-purpose Redis™ client. More .NET Redis™ clients can be found in the C# section of the Redis Clients™ page.
There are several ways to install this package:
- With the .NET CLI:
dotnet add package StackExchange.Redis
With the package manager console:
PM> Install-Package StackExchange.Redis
- With the NuGet GUI in Visual Studio
To connect your application, use one of the following commands:
using StackExchange.Redis;
using System;
using System.Linq;
using System.Net.Security;
using System.Security.Cryptography.X509Certificates;
using System.Threading.Tasks;
public class RedisConnectorHelper
{
const string PATH_TO_CERT_FILE = "c:\\PATH\\TO\\CAcert.pem";
static RedisConnectorHelper()
{
RedisConnectorHelper.lazyConnection = new Lazy<ConnectionMultiplexer>(() =>
{
var configurationOptions = new ConfigurationOptions
{
EndPoints = { "<YOUR_HOSTNAME>:6379” },
Password = "<YOUR_PASSWORD>",
Ssl = true
};
configurationOptions.CertificateValidation += CheckServerCertificate;
return ConnectionMultiplexer.Connect(configurationOptions);
});
}
private static bool CheckServerCertificate(object sender, X509Certificate certificate,
X509Chain chain, SslPolicyErrors sslPolicyErrors)
{
if (sslPolicyErrors == SslPolicyErrors.RemoteCertificateChainErrors)
{
// check that the untrusted ca is in the chain
var ca = new X509Certificate2(PATH_TO_CERT_FILE);
var caFound = chain.ChainElements
.Cast<X509ChainElement>()
.Any(x => x.Certificate.Thumbprint == ca.Thumbprint);
return caFound;
}
return false;
}
private static Lazy<ConnectionMultiplexer> lazyConnection;
public static ConnectionMultiplexer Connection
{
get
{
return lazyConnection.Value;
}
}
}
class Program
{
static void Main(string[] args)
{
var program = new Program();
var db = RedisConnectorHelper.Connection.GetDatabase();
db.StringSet("hello", "world");
Console.WriteLine(db.StringGet("hello"));
}
}
using StackExchange.Redis;
using System;
using System.Threading.Tasks;
public class RedisConnectorHelper
{
static RedisConnectorHelper()
{
RedisConnectorHelper.lazyConnection = new Lazy<ConnectionMultiplexer>(() =>
{
return ConnectionMultiplexer.Connect(
new ConfigurationOptions
{
EndPoints = { "<YOUR_HOSTNAME>:6379" },
Password = "<YOUR_PASSWORD>"
});
});
}
private static Lazy<ConnectionMultiplexer> lazyConnection;
public static ConnectionMultiplexer Connection
{
get
{
return lazyConnection.Value;
}
}
}
class Program
{
static void Main(string[] args)
{
var program = new Program();
var db = RedisConnectorHelper.Connection.GetDatabase();
db.StringSet("hello", "world");
Console.WriteLine(db.StringGet("hello"));
}
}
Updated 2 months ago