PHP MySQL Connection
Easily connect to your MySQL hosting deployments at ScaleGrid from PHP applications to optimize your MySQL management in the cloud.
How to Connect to MySQL from the PHP Application
- Install php and php-mysql packages
- Get the following MySQL deployment information from your cluster details page:
a. Username
b. Password
c. Hostname
d. Port (default 3306)
e. SSL certificate (if deployment has SSL)
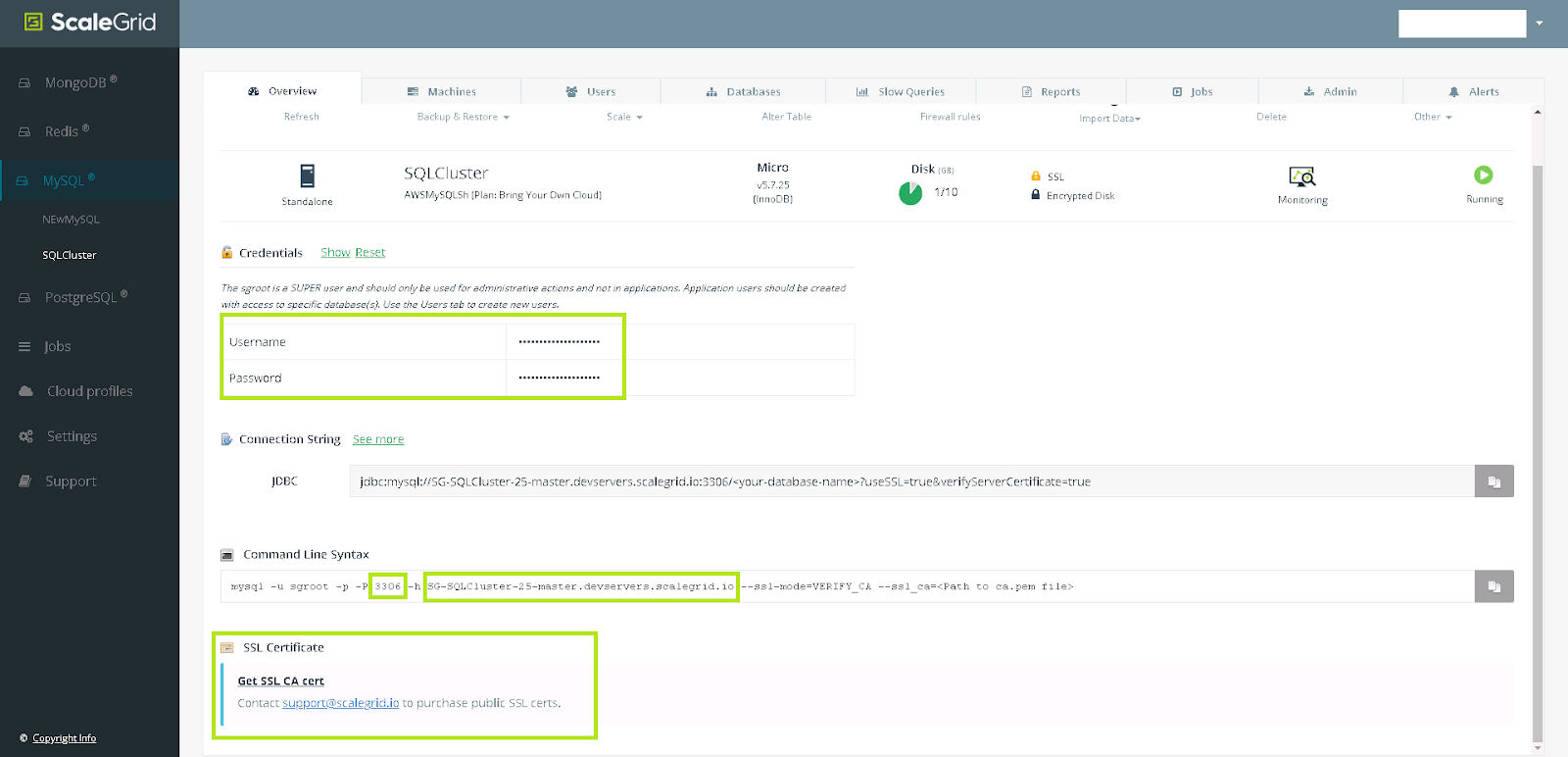
- Run the following code to connect to your MySQL deployment after you enter the information from step 2.
3a. Sample code to connect to MySQL without SSL:
[root@centos]# cat first.php
<?php
$servername = "SG-myssl-32702.servers.mongodirector.com";
$username = "t";
$password = "Test!123456";
// Create connection
$conn = new mysqli($servername, $username, $password);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$res = mysqli_query($conn, 'SHOW databases');
print_r(mysqli_fetch_row($res));
mysqli_close($conn);
?>
3b. Sample code to connect to MySQL with SSL:
Download your SSL certificate as shown in step 2 and save it as ca.pem file in the same directory as your PHP file.
[root@centos]# cat second.php
<?php
$servername = "SG-myssl-32702.servers.mongodirector.com";
$username = "t";
$password = "Test!123456";
$conn=mysqli_init();
mysqli_ssl_set($conn, NULL, NULL, 'ca.pem', NULL, '');
if (!mysqli_real_connect($conn, $servername, $username, $password )) { die("Connection failed"); }
$res = mysqli_query($conn, 'SHOW databases');
print_r(mysqli_fetch_row($res));
mysqli_close($conn);
?>
- Run the code:
4a. To run the PHP script to connect to MySQL without SSL:
[root@centos]# php first.php
Array
(
[0] => information_schema
)
4b. To run the PHP script to connect to MySQL with SSL:
[root@centos]# php second.php
Array
(
[0] => information_schema
)
Your PHP application is now connected to your MySQL deployment!
If you have any questions, please reach out to us at [email protected].
Updated over 1 year ago